mirror of
https://review.coreboot.org/flashrom.git
synced 2025-04-26 22:52:34 +02:00
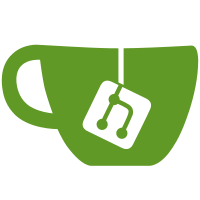
This adds a build-time option to automatically generate a list of authors from git history, and includes it in the documentation by reading the output from git in a Sphinx extension. When git isn't available or the project source doesn't appear to be a git checkout, the list is not generated and gracefully replaced with a message explaining its absence. Change-Id: I1e9634a90e84262aafd80590deba9875f4b71a3c Signed-off-by: Peter Marheine <pmarheine@chromium.org> Reviewed-on: https://review.coreboot.org/c/flashrom/+/86350 Tested-by: build bot (Jenkins) <no-reply@coreboot.org> Reviewed-by: Anastasia Klimchuk <aklm@chromium.org>
83 lines
2.5 KiB
Python
83 lines
2.5 KiB
Python
from docutils import nodes
|
|
from pathlib import Path
|
|
from typing import TYPE_CHECKING
|
|
|
|
from sphinx.application import Sphinx
|
|
from sphinx.util.docutils import SphinxDirective
|
|
|
|
if TYPE_CHECKING:
|
|
from sphinx.util.typing import ExtensionMetadata
|
|
|
|
|
|
class FlashromAuthorsDirective(SphinxDirective):
|
|
required_arguments = 1
|
|
has_content = True
|
|
|
|
def make_table(self, list_file: Path):
|
|
body = nodes.tbody()
|
|
with list_file.open("r") as f:
|
|
for line in f:
|
|
count, _, name = line.strip().partition("\t")
|
|
|
|
body += nodes.row(
|
|
"",
|
|
nodes.entry("", nodes.paragraph(text=name)),
|
|
nodes.entry("", nodes.paragraph(text=count)),
|
|
)
|
|
|
|
return nodes.table(
|
|
"",
|
|
nodes.tgroup(
|
|
"",
|
|
nodes.colspec(colname="name"),
|
|
nodes.colspec(colname="count"),
|
|
nodes.thead(
|
|
"",
|
|
nodes.row(
|
|
"",
|
|
nodes.entry("", nodes.paragraph(text="Name")),
|
|
nodes.entry("", nodes.paragraph(text="Number of changes")),
|
|
),
|
|
),
|
|
body,
|
|
cols=2,
|
|
),
|
|
)
|
|
|
|
def make_placeholder(self, contents):
|
|
return nodes.admonition(
|
|
"",
|
|
nodes.title("", text="List not available"),
|
|
*contents,
|
|
)
|
|
|
|
def run(self) -> list[nodes.Node]:
|
|
config = self.config.flashrom_authors_list_files
|
|
|
|
source_name = self.arguments[0]
|
|
list_file = (config or {}).get(source_name)
|
|
if list_file is None:
|
|
if config is not None:
|
|
available_names = ','.join(config.keys())
|
|
raise self.error(
|
|
'Undefined authors list file: "{}" (available names: {})'.format(
|
|
source_name, available_names
|
|
)
|
|
)
|
|
container = nodes.Element()
|
|
self.state.nested_parse(self.content, 0, container)
|
|
return [self.make_placeholder(container.children)]
|
|
else:
|
|
return [self.make_table(Path(list_file))]
|
|
|
|
|
|
def setup(app: Sphinx) -> 'ExtensionMetadata':
|
|
app.add_config_value(
|
|
"flashrom_authors_list_files", default=None, rebuild="html", types=[dict]
|
|
)
|
|
app.add_directive("flashrom-authors", FlashromAuthorsDirective)
|
|
|
|
return {
|
|
"version": "1",
|
|
}
|