mirror of
https://review.coreboot.org/flashrom.git
synced 2025-07-18 22:10:53 +02:00
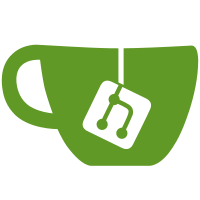
And even when it checks if the erase worked, the result of that check is often ignored. Convert all erase functions and actually check return codes almost everywhere. Check inside all erase_* routines if erase worked, not outside. erase_sector_jedec and erase_block_jedec have changed prototypes to enable erase checking. Uwe successfully tested LPC on an CK804 box and SPI on some SB600 box. Corresponding to flashrom svn r595. Signed-off-by: Carl-Daniel Hailfinger <c-d.hailfinger.devel.2006@gmx.net> Signed-off-by: Urja Rannikko <urjaman@gmail.com> Acked-by: Uwe Hermann <uwe@hermann-uwe.de>
97 lines
2.6 KiB
C
97 lines
2.6 KiB
C
/*
|
|
* This file is part of the flashrom project.
|
|
*
|
|
* Copyright (C) 2008 Peter Stuge <peter@stuge.se>
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA
|
|
*/
|
|
|
|
#include "flash.h"
|
|
|
|
int probe_w39v040c(struct flashchip *flash)
|
|
{
|
|
chipaddr bios = flash->virtual_memory;
|
|
uint8_t id1, id2, lock;
|
|
|
|
chip_writeb(0xAA, bios + 0x5555);
|
|
programmer_delay(10);
|
|
chip_writeb(0x55, bios + 0x2AAA);
|
|
programmer_delay(10);
|
|
chip_writeb(0x90, bios + 0x5555);
|
|
programmer_delay(10);
|
|
|
|
id1 = chip_readb(bios);
|
|
id2 = chip_readb(bios + 1);
|
|
lock = chip_readb(bios + 0xfff2);
|
|
|
|
chip_writeb(0xAA, bios + 0x5555);
|
|
programmer_delay(10);
|
|
chip_writeb(0x55, bios + 0x2AAA);
|
|
programmer_delay(10);
|
|
chip_writeb(0xF0, bios + 0x5555);
|
|
programmer_delay(40);
|
|
|
|
printf_debug("%s: id1 0x%02x, id2 0x%02x", __func__, id1, id2);
|
|
if (!oddparity(id1))
|
|
printf_debug(", id1 parity violation");
|
|
printf_debug("\n");
|
|
if (flash->manufacture_id == id1 && flash->model_id == id2) {
|
|
printf("%s: Boot block #TBL is %slocked, rest of chip #WP is %slocked.\n",
|
|
__func__, lock & 0x4 ? "" : "un", lock & 0x8 ? "" : "un");
|
|
return 1;
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
|
|
int erase_w39v040c(struct flashchip *flash)
|
|
{
|
|
int i;
|
|
unsigned int total_size = flash->total_size * 1024;
|
|
|
|
for (i = 0; i < total_size; i += flash->page_size) {
|
|
if (erase_sector_jedec(flash, i, flash->page_size)) {
|
|
fprintf(stderr, "ERASE FAILED!\n");
|
|
return -1;
|
|
}
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
|
|
int write_w39v040c(struct flashchip *flash, uint8_t *buf)
|
|
{
|
|
int i;
|
|
int total_size = flash->total_size * 1024;
|
|
int page_size = flash->page_size;
|
|
chipaddr bios = flash->virtual_memory;
|
|
|
|
if (flash->erase(flash)) {
|
|
fprintf(stderr, "ERASE FAILED!\n");
|
|
return -1;
|
|
}
|
|
|
|
printf("Programming page: ");
|
|
for (i = 0; i < total_size / page_size; i++) {
|
|
printf("%04d at address: 0x%08x", i, i * page_size);
|
|
write_sector_jedec(bios, buf + i * page_size,
|
|
bios + i * page_size, page_size);
|
|
printf("\b\b\b\b\b\b\b\b\b\b\b\b\b\b\b\b\b\b\b\b\b\b\b\b\b\b\b");
|
|
}
|
|
printf("\n");
|
|
|
|
return 0;
|
|
}
|