mirror of
https://review.coreboot.org/flashrom.git
synced 2025-07-12 02:50:47 +02:00
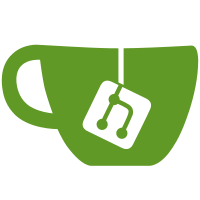
And even when it checks if the erase worked, the result of that check is often ignored. Convert all erase functions and actually check return codes almost everywhere. Check inside all erase_* routines if erase worked, not outside. erase_sector_jedec and erase_block_jedec have changed prototypes to enable erase checking. Uwe successfully tested LPC on an CK804 box and SPI on some SB600 box. Corresponding to flashrom svn r595. Signed-off-by: Carl-Daniel Hailfinger <c-d.hailfinger.devel.2006@gmx.net> Signed-off-by: Urja Rannikko <urjaman@gmail.com> Acked-by: Uwe Hermann <uwe@hermann-uwe.de>
73 lines
2.1 KiB
C
73 lines
2.1 KiB
C
/*
|
|
* This file is part of the flashrom project.
|
|
*
|
|
* Copyright (C) 2000 Silicon Integrated System Corporation
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA
|
|
*/
|
|
|
|
#include "flash.h"
|
|
|
|
int erase_49lf040(struct flashchip *flash)
|
|
{
|
|
int i;
|
|
int total_size = flash->total_size * 1024;
|
|
int page_size = flash->page_size;
|
|
|
|
for (i = 0; i < total_size / page_size; i++) {
|
|
/* Chip erase only works in parallel programming mode
|
|
* for the 49lf040. Use sector-erase instead */
|
|
if (erase_sector_jedec(flash, i * page_size, page_size)) {
|
|
fprintf(stderr, "ERASE FAILED!\n");
|
|
return -1;
|
|
}
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
|
|
int write_49lf040(struct flashchip *flash, uint8_t *buf)
|
|
{
|
|
int i;
|
|
int total_size = flash->total_size * 1024;
|
|
int page_size = flash->page_size;
|
|
chipaddr bios = flash->virtual_memory;
|
|
|
|
printf("Programming page: ");
|
|
for (i = 0; i < total_size / page_size; i++) {
|
|
/* erase the page before programming
|
|
* Chip erase only works in parallel programming mode
|
|
* for the 49lf040. Use sector-erase instead */
|
|
if (erase_sector_jedec(flash, i * page_size, page_size)) {
|
|
fprintf(stderr, "ERASE FAILED!\n");
|
|
return -1;
|
|
}
|
|
|
|
/* write to the sector */
|
|
if (i % 10 == 0)
|
|
printf("%04d at address: 0x%08x ", i, i * page_size);
|
|
|
|
write_sector_jedec(bios, buf + i * page_size,
|
|
bios + i * page_size, page_size);
|
|
|
|
if (i % 10 == 0)
|
|
printf("\b\b\b\b\b\b\b\b\b\b\b\b\b\b\b\b\b\b\b\b\b\b\b\b\b\b\b\b");
|
|
fflush(stdout);
|
|
}
|
|
printf("\n");
|
|
|
|
return 0;
|
|
}
|