mirror of
https://review.coreboot.org/flashrom.git
synced 2025-04-27 15:12:36 +02:00
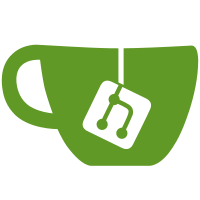
Allow specialisation in opaque masters, such as ichspi hwseq, to write to status registers. Also update the dispatch logic in libflashrom to call wp code when status register access functions are provided by an opaque master. BUG=none BRANCH=none TEST=flashrom --wp-status on AMD and Intel DUTs Change-Id: I3ab0d7f5f48338c8ecb118a69651c203fbc516ac Signed-off-by: Edward O'Callaghan <quasisec@google.com> Signed-off-by: Nikolai Artemiev <nartemiev@google.com> Co-Authored-by: Nikolai Artemiev <nartemiev@google.com> Reviewed-on: https://review.coreboot.org/c/flashrom/+/64375 Reviewed-by: Anastasia Klimchuk <aklm@chromium.org> Tested-by: build bot (Jenkins) <no-reply@coreboot.org>
93 lines
2.4 KiB
C
93 lines
2.4 KiB
C
/*
|
|
* This file is part of the flashrom project.
|
|
*
|
|
* Copyright (C) 2010 Google Inc.
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
*/
|
|
|
|
#ifndef __WRITEPROTECT_H__
|
|
#define __WRITEPROTECT_H__ 1
|
|
|
|
#include <stdint.h>
|
|
#include <stdbool.h>
|
|
#include <stddef.h>
|
|
|
|
#include "libflashrom.h"
|
|
|
|
#define MAX_BP_BITS 4
|
|
|
|
/* Chip protection range: start address and length. */
|
|
struct wp_range {
|
|
size_t start, len;
|
|
};
|
|
|
|
/* Generic description of a chip's write protection configuration. */
|
|
struct flashrom_wp_cfg {
|
|
enum flashrom_wp_mode mode;
|
|
struct wp_range range;
|
|
};
|
|
|
|
/* Collection of multiple write protection ranges. */
|
|
struct flashrom_wp_ranges {
|
|
struct wp_range *ranges;
|
|
size_t count;
|
|
};
|
|
|
|
/*
|
|
* Description of a chip's write protection configuration.
|
|
*
|
|
* It allows most WP code to store and manipulate a chip's configuration
|
|
* without knowing the exact layout of bits in the chip's status registers.
|
|
*/
|
|
struct wp_bits {
|
|
/* Status register protection bit (SRP) */
|
|
bool srp_bit_present;
|
|
uint8_t srp;
|
|
|
|
/* Status register lock bit (SRL) */
|
|
bool srl_bit_present;
|
|
uint8_t srl;
|
|
|
|
/* Complement bit (CMP) */
|
|
bool cmp_bit_present;
|
|
uint8_t cmp;
|
|
|
|
/* Sector/block protection bit (SEC) */
|
|
bool sec_bit_present;
|
|
uint8_t sec;
|
|
|
|
/* Top/bottom protection bit (TB) */
|
|
bool tb_bit_present;
|
|
uint8_t tb;
|
|
|
|
/* Block protection bits (BP) */
|
|
size_t bp_bit_count;
|
|
uint8_t bp[MAX_BP_BITS];
|
|
};
|
|
|
|
struct flashrom_flashctx;
|
|
|
|
/* Write WP configuration to the chip */
|
|
enum flashrom_wp_result wp_write_cfg(struct flashrom_flashctx *, const struct flashrom_wp_cfg *);
|
|
|
|
/* Read WP configuration from the chip */
|
|
enum flashrom_wp_result wp_read_cfg(struct flashrom_wp_cfg *, struct flashrom_flashctx *);
|
|
|
|
/* Get a list of protection ranges supported by the chip */
|
|
enum flashrom_wp_result wp_get_available_ranges(struct flashrom_wp_ranges **, struct flashrom_flashctx *);
|
|
|
|
/* Checks if writeprotect functions can be used with the current flash/programmer */
|
|
bool wp_operations_available(struct flashrom_flashctx *);
|
|
|
|
#endif /* !__WRITEPROTECT_H__ */
|