mirror of
https://review.coreboot.org/flashrom.git
synced 2025-07-02 06:23:18 +02:00
tests: assert pathname and flags when calling open()
With this change the wrappers for mock and friends are able to take an optional io_mock_fallback_open_state struct to assert expected pathnames and flags whenever an open operation is called. Based partially on https://review.coreboot.org/c/flashrom/+/62319/5 BUG=b:227404721,b:217629892,b:215255210 TEST=./test_build.sh; FEATURES=test emerge-amd64-generic flashrom BRANCH=none Signed-off-by: Daniel Campello <campello@chromium.org> Co-Author: Edward O'Callaghan <quasisec@google.com> Change-Id: Ib46ca5b854c8453ec02ae09f3151cd4d25f988eb Reviewed-on: https://review.coreboot.org/c/flashrom/+/63227 Tested-by: build bot (Jenkins) <no-reply@coreboot.org> Reviewed-by: Anastasia Klimchuk <aklm@chromium.org>
This commit is contained in:
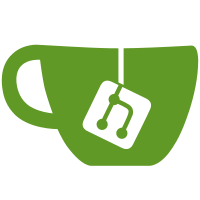
committed by
Anastasia Klimchuk
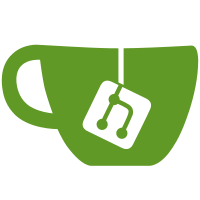
parent
9454336970
commit
885fb2e82b
@ -14,12 +14,15 @@
|
||||
* GNU General Public License for more details.
|
||||
*/
|
||||
|
||||
#include <include/test.h>
|
||||
#include "io_mock.h"
|
||||
|
||||
static const struct io_mock *current_io = NULL;
|
||||
|
||||
void io_mock_register(const struct io_mock *io)
|
||||
{
|
||||
/* A test can either register its own mock open function or fallback_open_state. */
|
||||
assert_true(io == NULL || io->open == NULL || io->fallback_open_state == NULL);
|
||||
current_io = io;
|
||||
}
|
||||
|
||||
|
@ -60,6 +60,15 @@ struct pci_dev {
|
||||
/* Always return success for tests. */
|
||||
#define S_ISREG(x) 0
|
||||
|
||||
/* Maximum number of open calls to mock. This number is arbitrary. */
|
||||
#define MAX_MOCK_OPEN 4
|
||||
|
||||
struct io_mock_fallback_open_state {
|
||||
unsigned int noc;
|
||||
const char *paths[MAX_MOCK_OPEN];
|
||||
int flags[MAX_MOCK_OPEN];
|
||||
};
|
||||
|
||||
struct io_mock {
|
||||
void *state;
|
||||
|
||||
@ -105,6 +114,12 @@ struct io_mock {
|
||||
size_t (*fread)(void *state, void *buf, size_t size, size_t len, FILE *fp);
|
||||
int (*fprintf)(void *state, FILE *fp, const char *fmt, va_list args);
|
||||
int (*fclose)(void *state, FILE *fp);
|
||||
|
||||
/*
|
||||
* An alternative to custom open mock. A test can either register its
|
||||
* own mock open function or fallback_open_state.
|
||||
*/
|
||||
struct io_mock_fallback_open_state *fallback_open_state;
|
||||
};
|
||||
|
||||
void io_mock_register(const struct io_mock *io);
|
||||
|
@ -370,13 +370,6 @@ void linux_spi_probe_lifecycle_test_success(void **state)
|
||||
#endif
|
||||
}
|
||||
|
||||
static int realtek_mst_open(void *state, const char *pathname, int flags)
|
||||
{
|
||||
assert_string_equal(pathname, "/dev/i2c-254");
|
||||
assert_int_equal(flags & O_RDWR, O_RDWR);
|
||||
return MOCK_FD;
|
||||
}
|
||||
|
||||
static int realtek_mst_ioctl(void *state, int fd, unsigned long request, va_list args)
|
||||
{
|
||||
assert_int_equal(fd, MOCK_FD);
|
||||
@ -406,11 +399,16 @@ static int realtek_mst_write(void *state, int fd, const void *buf, size_t sz)
|
||||
void realtek_mst_basic_lifecycle_test_success(void **state)
|
||||
{
|
||||
#if CONFIG_REALTEK_MST_I2C_SPI == 1
|
||||
static struct io_mock_fallback_open_state realtek_mst_fallback_open_state = {
|
||||
.noc = 0,
|
||||
.paths = { "/dev/i2c-254", NULL },
|
||||
.flags = { O_RDWR },
|
||||
};
|
||||
const struct io_mock realtek_mst_io = {
|
||||
.open = realtek_mst_open,
|
||||
.ioctl = realtek_mst_ioctl,
|
||||
.read = realtek_mst_read,
|
||||
.write = realtek_mst_write,
|
||||
.fallback_open_state = &realtek_mst_fallback_open_state,
|
||||
};
|
||||
io_mock_register(&realtek_mst_io);
|
||||
|
||||
|
@ -74,28 +74,43 @@ uint8_t __wrap_sio_read(uint16_t port, uint8_t reg)
|
||||
return (uint8_t)mock();
|
||||
}
|
||||
|
||||
static int mock_open(const char *pathname, int flags)
|
||||
{
|
||||
if (get_io() && get_io()->open)
|
||||
return get_io()->open(get_io()->state, pathname, flags);
|
||||
|
||||
if (get_io() && get_io()->fallback_open_state) {
|
||||
struct io_mock_fallback_open_state *io_state;
|
||||
unsigned int open_state_flags;
|
||||
|
||||
io_state = get_io()->fallback_open_state;
|
||||
assert_true(io_state->noc < MAX_MOCK_OPEN);
|
||||
assert_non_null(io_state->paths[io_state->noc]);
|
||||
assert_string_equal(pathname, io_state->paths[io_state->noc]);
|
||||
open_state_flags = io_state->flags[io_state->noc];
|
||||
assert_int_equal(flags & open_state_flags, open_state_flags);
|
||||
io_state->noc++; // proceed to the next path upon next call.
|
||||
}
|
||||
|
||||
return MOCK_FD;
|
||||
}
|
||||
|
||||
int __wrap_open(const char *pathname, int flags)
|
||||
{
|
||||
LOG_ME;
|
||||
if (get_io() && get_io()->open)
|
||||
return get_io()->open(get_io()->state, pathname, flags);
|
||||
return MOCK_FD;
|
||||
return mock_open(pathname, flags);
|
||||
}
|
||||
|
||||
int __wrap_open64(const char *pathname, int flags)
|
||||
{
|
||||
LOG_ME;
|
||||
if (get_io() && get_io()->open)
|
||||
return get_io()->open(get_io()->state, pathname, flags);
|
||||
return MOCK_FD;
|
||||
return mock_open(pathname, flags);
|
||||
}
|
||||
|
||||
int __wrap___open64_2(const char *pathname, int flags)
|
||||
{
|
||||
LOG_ME;
|
||||
if (get_io() && get_io()->open)
|
||||
return get_io()->open(get_io()->state, pathname, flags);
|
||||
return MOCK_FD;
|
||||
return mock_open(pathname, flags);
|
||||
}
|
||||
|
||||
int __wrap_ioctl(int fd, unsigned long int request, ...)
|
||||
|
Reference in New Issue
Block a user